The enclosed efs template can be used to create virtually any formula.
All the most relevant and commonly used parts of a script are identified and commented. For detailed explanations on these parts and instructions on how to use them refer to the Guide to developing eSignal Indicators
Copy of this template can also be downloaded from here
Following is the same template set up as a complete formula. This efs uses a moving average to color the price bars in blue if the Close is above the average or in red if below. Results are shown in the image that follows the code.
All the most relevant and commonly used parts of a script are identified and commented. For detailed explanations on these parts and instructions on how to use them refer to the Guide to developing eSignal Indicators
Copy of this template can also be downloaded from here
PHP Code:
//Alexis C. Montenegro © October 2005
/****************************** preMain function **************************************/
function preMain(){
setPriceStudy(true/false);//select if price (true) OR non-price (false) study
setStudyTitle("");//add the study title
//following statements define the default properties of the returned item
setCursorLabelName("label name",0);//cursor window label name
setDefaultBarFgColor(Color.blue,0);//plot color
setPlotType(PLOTTYPE_LINE,0);//plot type
setDefaultBarThickness(1,0);//plot thickness
//if returning two items uncomment the following lines
//setCursorLabelName("label name",1);
//setDefaultBarFgColor(Color.red,1);
//setPlotType(PLOTTYPE_LINE,1);
//setDefaultBarThickness(1,1);
//if returning more than two items add the required statements
//setCursorLabelName("label name",2);
//etc
//if painting the price bars uncomment the following lines
//setColorPriceBars(true);
//setDefaultPriceBarColor(Color.black);
}
/******************************* end preMain function **********************************/
/******* declare global variables here **********/
//for example var myGlobalVar = null;
/*********** end global variables ***************/
/******************************* main function *****************************************/
function main(){
/************** declare local variables here ***********************/
//for example var myLocalVar = 0;
/****************** end local variables ****************************/
/************ initialize global variables here *********************/
//for example if(myGlobalVar==null) myGlobalVar = sma(10);
/*************** end intialization variables **********************/
/*************** insert your main code here ********************/
/*** if part of the code needs to be computed only on a new bar ****/
/*** insert code inside the BARSTATE_NEWBAR condition ****/
if(getBarState()==BARSTATE_NEWBAR){
}
/******************* end BARSTATE_NEWBAR condition *****************/
/********************* end main code here **************************/
/****************** insert your return here ***********************/
//if only one item use return (myVar);
//if more than one item use return new Array (myVar1, myVar2, etc);
/********************** end return *********************************/
}
/******************************* end main function **************************************/
Following is the same template set up as a complete formula. This efs uses a moving average to color the price bars in blue if the Close is above the average or in red if below. Results are shown in the image that follows the code.
PHP Code:
//Alexis C. Montenegro © October 2005
/****************************** preMain function **************************************/
function preMain(){
setPriceStudy(true);//select if price (true) OR non-price (false) study
setStudyTitle("MA Price Bars");//add the study title
//following statements define the default properties of the returned item
setCursorLabelName("MA",0);//cursor window label name
setDefaultBarFgColor(Color.blue,0);//plot color
setPlotType(PLOTTYPE_LINE,0);//plot type
setDefaultBarThickness(1,0);//plot thickness
//if returning two items uncomment the following lines
//setCursorLabelName("label name",1);
//setDefaultBarFgColor(Color.red,1);
//setPlotType(PLOTTYPE_LINE,1);
//setDefaultBarThickness(1,1);
//if returning more than two items add the required statements
//setCursorLabelName("label name",2);
//etc
//if painting the price bars uncomment the following lines
setColorPriceBars(true);
setDefaultPriceBarColor(Color.black);
}
/******************************* end preMain function *********************************/
/******* declare global variables here **********/
//for example var myGlobalVar = null;
var myStudy = null;
/*********** end global variables ***************/
/******************************* main function ****************************************/
function main(){
/************** declare local variables here ***********************/
//for example var myLocalVar = 0;
var myMA = 0;
var Close = close(0);
/****************** end local variables ****************************/
/************ initialize global variables here *********************/
//for example if(myGlobalVar==null) myGlobalVar = sma(10);
if (myStudy == null) myStudy = sma(10);
/*************** end intialization variables **********************/
/*************** insert your main code here ********************/
myMA = myStudy.getValue(0);
if (myMA == null || Close == null) {
return;
}
/*** if part of the code needs to be computed only on a new bar ****/
/*** insert code inside the BARSTATE_NEWBAR condition ****/
if(getBarState()==BARSTATE_NEWBAR){
}
/******************* end BARSTATE_NEWBAR condition *****************/
if (Close > myMA){
setPriceBarColor(Color.blue);
} else if (Close < myMA){
setPriceBarColor(Color.red);
}
/********************* end main code here **************************/
/****************** insert your return here ***********************/
//if only one item use return (myVar);
//if more than one item use return new Array (myVar1, myVar2, etc);
return myMA;
/********************** end return *********************************/
}
/******************************* end main function *************************************/
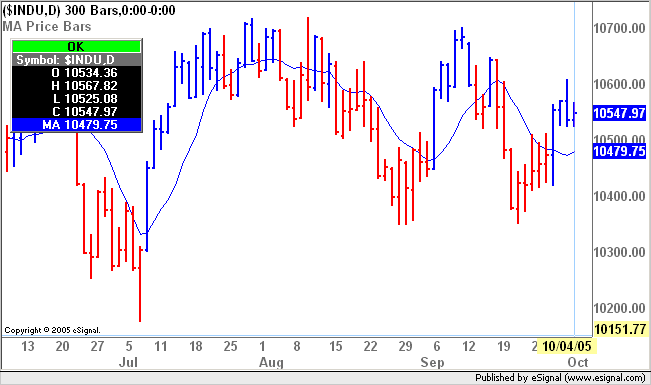
Comment