The attached efs will calculate the overnight high and low of a security.
In Edit Studies you can set the Start time (default is 16:15) and End time (default 9:30). A third option - set On by default - extends the overnight high/low lines into the current day (shown in the enclosed image)
Alex
In Edit Studies you can set the Start time (default is 16:15) and End time (default 9:30). A third option - set On by default - extends the overnight high/low lines into the current day (shown in the enclosed image)
Alex
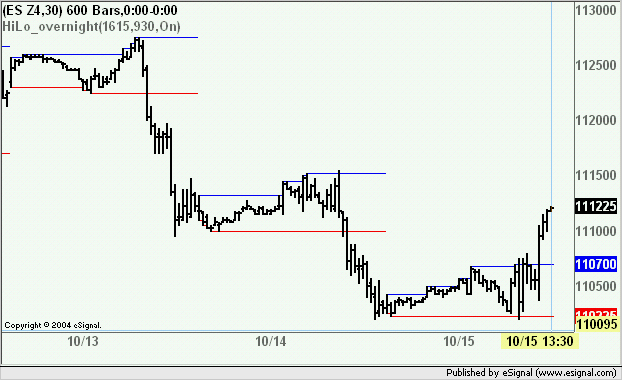
Comment