File Name: EquityCurve_MA.efs
Description:
This study is based on the December 2006 Stock Trading System Lab article, Trading the Equity Curve, by Volker Knapp.
Formula Parameters:
KAMA Periods 10
KAMA Fast Length 2
KAMA Slow Length 30
Commission per Trade: 8
Slippage per Trade: 0.002 (0.2%)
Lot Size: 100
Bollinger Band Periods: 38
Bollinger Band Standard Deviations: 3
Fast SMA Length: 3
Slow SMA Length: 10
Notes:
The related article is copyrighted material. If you are not a subscriber of Active Trader Magazine, please visit www.activetradermag.com.
Download File:
EquityCurve_MA.efs
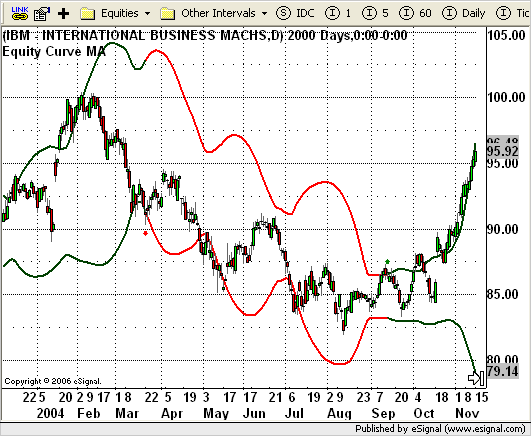
EFS Code:
Description:
This study is based on the December 2006 Stock Trading System Lab article, Trading the Equity Curve, by Volker Knapp.
Formula Parameters:
KAMA Periods 10
KAMA Fast Length 2
KAMA Slow Length 30
Commission per Trade: 8
Slippage per Trade: 0.002 (0.2%)
Lot Size: 100
Bollinger Band Periods: 38
Bollinger Band Standard Deviations: 3
Fast SMA Length: 3
Slow SMA Length: 10
Notes:
The related article is copyrighted material. If you are not a subscriber of Active Trader Magazine, please visit www.activetradermag.com.
Download File:
EquityCurve_MA.efs
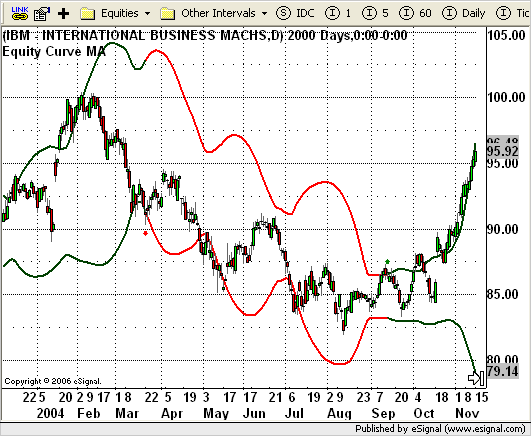
EFS Code:
PHP Code:
/***************************************
Provided By : eSignal (c) Copyright 2006
Description: Trading the equity curve
by Volker Knapp
Version 1.0 11/17/2006
Notes:
* Dec 2006 Issue of Active Trader Magazine
* Study requires version 8.0 or later.
Formula Parameters: Default:
KAMA Periods 10
KAMA Fast Length 2
KAMA Slow Length 30
Commission per Trade 8
Slippage per Trade 0.002 (0.2%)
Lot Size 100
Bollinger Band Periods 38
Bollinger Band Standard Deviations 3
Fast SMA Length 3
Slow SMA Length 10
***************************************/
function preMain() {
setPriceStudy(true);
setStudyTitle("Equity Curve MA");
setShowTitleParameters(false);
setCursorLabelName("Upper BB", 0);
setCursorLabelName("Lower BB", 1);
setCursorLabelName("Equity Fast MA", 2);
setCursorLabelName("Equity Slow MA", 3);
setDefaultBarFgColor(Color.grey, 0);
setDefaultBarFgColor(Color.grey, 1);
setDefaultBarFgColor(Color.red, 2);
setDefaultBarFgColor(Color.green, 3);
setDefaultBarThickness(2, 0);
setDefaultBarThickness(2, 1);
// KAMA parameters
var fp1 = new FunctionParameter( "Period", FunctionParameter.NUMBER);
fp1.setName( "KAMA Periods" );
fp1.setLowerLimit( 5 );
fp1.setUpperLimit( 125 );
fp1.setDefault( 10 );
var fp2 = new FunctionParameter( "Fast", FunctionParameter.NUMBER);
fp2.setName( "KAMA Fast Length" );
fp2.setLowerLimit( 1 );
fp2.setUpperLimit( 125 );
fp2.setDefault( 2 );
var fp3 = new FunctionParameter( "Slow", FunctionParameter.NUMBER);
fp3.setName( "KAMA Slow Length" );
fp3.setLowerLimit( 1 );
fp3.setUpperLimit( 125 );
fp3.setDefault( 30 );
var fp4 = new FunctionParameter( "Commission", FunctionParameter.NUMBER);
fp4.setName( "Commission per Trade" );
fp4.setLowerLimit( 0 );
fp4.setDefault( 8 );
var fp5 = new FunctionParameter( "Slippage", FunctionParameter.NUMBER);
fp5.setName( "Slippage per Trade" );
fp5.setLowerLimit( 0 );
fp5.setDefault( 0.002 );
var fp5b = new FunctionParameter( "Lot", FunctionParameter.NUMBER);
fp5b.setName( "Lot Size" );
fp5b.setLowerLimit( 1 );
fp5b.setDefault( 100 );
var fp6 = new FunctionParameter( "BBPeriods", FunctionParameter.NUMBER);
fp6.setName( "Bollinger Band Periods" );
fp6.setLowerLimit( 1 );
fp6.setDefault( 38 );
var fp7 = new FunctionParameter( "BBStdv", FunctionParameter.NUMBER);
fp7.setName( "Bollinger Band Standard Deviations" );
fp7.setLowerLimit( 0 );
fp7.setDefault( 3 );
var fp8 = new FunctionParameter( "SMA_fast", FunctionParameter.NUMBER);
fp8.setName( "Fast SMA Length " );
fp8.setLowerLimit( 0 );
fp8.setDefault( 3 );
var fp9 = new FunctionParameter( "SMA_slow", FunctionParameter.NUMBER);
fp9.setName( "Slow SMA Length " );
fp9.setLowerLimit( 0 );
fp9.setDefault( 10 );
}
// Global Variables
var bVersion = null; // Version flag
var bInit = false; // Initialization flag
var bBT = true; // Back Testing flag
// Basis Strategy Variables
var xUpper = null;
var xLower = null;
var xEquity = null; // Equity Series
var nEquity = 100000;
var nLot = 100;
var nEntry = null;
var nExit = null;
var nCom = 8*2; // Commission per trade
var nSlip = 0.002; // 0.2% Slippage per trade
var vPosition = 0; // 1=long, 0=flat, -1=short
// Strategy of Basis (B)
var xSMA_fast = null;
var xSMA_slow = null;
var vPositionB = 0; // 1=long, 0=flat, -1=short
// KAMA globals
var xKamaH = null;
var xKamaL = null;
var nKama = 0; // Kama value
var nKama_1 = 0; // previous bar's Kama value
var nFast = 0;
var nSlow = 0;
function main(Period, Fast, Slow, Commission, Slippage, Lot,
BBPeriods, BBStdv, SMA_fast, SMA_slow) {
if (bVersion == null) bVersion = verify();
if (bVersion == false) return;
var nState = getBarState();
if(bInit == false) {
xKamaH = efsInternal("kama", Period, Fast, Slow, high());
xKamaL = efsInternal("kama", Period, Fast, Slow, low());
xUpper = upperBB(BBPeriods, BBStdv, xKamaH);
xLower = lowerBB(BBPeriods, BBStdv, xKamaL);
// Basis Strategy for Paper trading equity curve needs to go
// into the getBasisEquity series function. Any existing strategy
// may be used in place of the current strategy.
xEquity = efsInternal("getBasisEquity", xUpper, xLower);
xSMA_fast = sma(SMA_fast, xEquity);
xSMA_slow = sma(SMA_slow, xEquity);
nCom = Commission * 2;
nSlip = Slippage;
nLot = Lot;
bInit = true;
}
if (nState == BARSTATE_NEWBAR) {
if (getCurrentBarIndex() == 0) bBT = false;
}
var nU = xUpper.getValue(-1);
var nL = xLower.getValue(-1);
var nSMA_fast_1 = xSMA_fast.getValue(-1);
var nSMA_slow_1 = xSMA_slow.getValue(-1);
if(nU == null || nL == null ||
nSMA_fast_1 == null || nSMA_slow_1 == null) return;
/***** Strategy of Basis (B) *****/
if (nState == BARSTATE_NEWBAR) {
if (nSMA_fast_1 > nSMA_slow_1) {
// Long Trade Signal
if (vPositionB != 1 && close(-1) > nU) {
drawShape(Shape.UPARROW, AboveBar1, Color.green, rawtime(0));
vPositionB = 1;
if (bBT == true) {
Strategy.doLong("Long", Strategy.MARKET, Strategy.THISBAR);
}
// Short Trade Signal
} else if (vPositionB != -1 && close(-1) < nL) {
drawShape(Shape.DOWNARROW, BelowBar1, Color.red, rawtime(0));
vPositionB = -1;
if (bBT == true) {
Strategy.doShort("Short", Strategy.MARKET, Strategy.THISBAR);
}
}
}
}
if(vPositionB == 1) {
setBarFgColor(Color.darkgreen, 0);
setBarFgColor(Color.darkgreen, 1);
} else if(vPositionB == -1) {
setBarFgColor(Color.red, 0);
setBarFgColor(Color.red, 1);
}
return new Array((xUpper.getValue(0)).toFixed(2)*1,
(xLower.getValue(0)).toFixed(2)*1,
(xSMA_fast.getValue(0)).toFixed(2),
(xSMA_slow.getValue(0)).toFixed(2));
}
function getBasisEquity(xU, xL) {
var nState = getBarState();
var nU = xU.getValue(-1);
var nL = xL.getValue(-1);
if (nState == BARSTATE_NEWBAR) {
// Long Trade Signal
if (vPosition != 1 && close(-1) > nU) {
nExit = open(0) + (nSlip * open(0));
if (vPosition == -1) {
nEquity += ((nEntry - nExit) * nLot) - nCom;
}
vPosition = 1;
nEntry = open(0) + (nSlip * open(0));
// Short Trade Signal
} else if (vPosition != -1 && close(-1) < nL) {
nExit = open(0) - (nSlip * open(0));
if (vPosition == 1) {
nEquity += ((nExit - nEntry) * nLot) - nCom;
}
vPosition = -1;
nEntry = open(0) - (nSlip * open(0));
}
}
return nEquity;
}
function kama(Period, Fast, Slow, xSource) {
/****************************
kama series modified from KAMA.efs by
Divergence Software, Inc.
[url]http://share.esignal.com/groupgo.jsp?groupid=114[/url]
****************************/
var x = 0;
var nNoise;
var nSignal;
var nSmooth;
if (bInit == false) {
nFast = 2 / ( Fast + 1 );
nSlow = 2 / ( Slow + 1 );
bInit = true;
}
if (getBarState() == BARSTATE_NEWBAR) {
nKama_1 = nKama;
}
if (xSource.getValue(-(Period+1)) == null) return close(0);
nSignal = Math.abs( xSource.getValue(0) - xSource.getValue(-Period) );
nNoise = 0;
while( x<Period ) {
nNoise += Math.abs( xSource.getValue(-x)-xSource.getValue(-(x+1)) );
x++;
}
if ( nNoise==0 ) nNoise = 1.0;
nSmooth = Math.pow( ( nSignal/nNoise ) * ( nFast - nSlow ) + nSlow , 2 );
nKama = nKama_1 + nSmooth * ( xSource.getValue(0) - nKama_1 );
return nKama;
}
function verify() {
var b = false;
if (getBuildNumber() < 779) {
drawTextAbsolute(5, 35, "This study requires version 8.0 or later.",
Color.white, Color.blue, Text.RELATIVETOBOTTOM|Text.RELATIVETOLEFT|Text.BOLD|Text.LEFT,
null, 13, "error");
drawTextAbsolute(5, 20, "Click HERE to upgrade.@URL=http://www.esignal.com/download/default.asp",
Color.white, Color.blue, Text.RELATIVETOBOTTOM|Text.RELATIVETOLEFT|Text.BOLD|Text.LEFT,
null, 13, "upgrade");
return b;
} else {
b = true;
}
return b;
}