File Name: LSMA_Channel.efs
Description:
Calculates the Least Square Moving Average (LSMA)
off the high and also off the low to create a channel.
Formula Parameters:
HiLength - Defines the length of the LSMA off the High. Default is 25.
LoLength - Defines the length of the LSMA off the Low. Default is 25.
HiOffset - Defines how offset the LSMA(High) is. Default is 0.
LoOffset - Defines how offset the LSMA(Low) is. Default is 0.
HiColor - Defines the color of the LSMA(High). Default is blue.
LoColor - Defines the color of the LSMA(Low). Default is red.
Download File:
LSMA_Channel.efs
EFS Code:
Description:
Calculates the Least Square Moving Average (LSMA)
off the high and also off the low to create a channel.
Formula Parameters:
HiLength - Defines the length of the LSMA off the High. Default is 25.
LoLength - Defines the length of the LSMA off the Low. Default is 25.
HiOffset - Defines how offset the LSMA(High) is. Default is 0.
LoOffset - Defines how offset the LSMA(Low) is. Default is 0.
HiColor - Defines the color of the LSMA(High). Default is blue.
LoColor - Defines the color of the LSMA(Low). Default is red.
Download File:
LSMA_Channel.efs
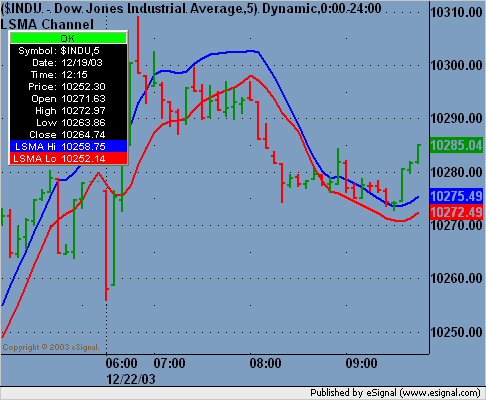
EFS Code:
PHP Code:
/*************************
Copyright © eSignal, 2003
**************************
Description: Calculates the Least Square Moving Average (LSMA)
off the high and also off the low to create a channel.
Version Control:
*/
function preMain()
{
setPriceStudy(true);
setStudyTitle("LSMA Channel");
setCursorLabelName("LSMA Hi",0);
setCursorLabelName("LSMA Lo",1);
setDefaultBarThickness(2,0);
setDefaultBarThickness(2,1);
var fp1 = new FunctionParameter("HiLength", FunctionParameter.NUMBER);
fp1.setName("Length of LSMA(High)");
fp1.setDefault(25);
var fp2 = new FunctionParameter("LoLength", FunctionParameter.NUMBER);
fp2.setName("Length of LSMA(Low)");
fp2.setDefault(25);
var fp3 = new FunctionParameter("HiOffset", FunctionParameter.NUMBER);
fp3.setName("Offset of LSMA(High)");
fp3.setDefault(0);
var fp4 = new FunctionParameter("LoOffset", FunctionParameter.NUMBER);
fp4.setName("Offset of LSMA(Low)");
fp4.setDefault(0);
var fp5 = new FunctionParameter("HiColor", FunctionParameter.COLOR);
fp5.setName("Color of LSMA(High)");
fp5.setDefault(Color.blue);
var fp6 = new FunctionParameter("LoColor", FunctionParameter.COLOR);
fp6.setName("Color of LSMA(Low)");
fp6.setDefault(Color.red);
}
var aHigh = null;
var aLow = null;
var aHiLSMA = null;
var aLoLSMA = null;
var vInit = false;
var LSMA_Array = new Array();
function main(HiLength,LoLength,HiOffset,LoOffset,HiColor,LoColor)
{
if (vInit == false) {
aHigh = new Array(HiLength);
aLow = new Array(LoLength);
aHiLSMA = new Array(HiLength);
aLoLSMA = new Array(LoLength);
setDefaultBarFgColor(HiColor,0);
setDefaultBarFgColor(LoColor,1);
preMain();
vInit = true;
}
vHigh = high();
vLow = low();
if (vHigh == null) return;
if (vLow == null) return;
if (getBarState() == BARSTATE_NEWBAR) {
if (aHigh[HiLength-1] != null) aHigh.pop();
aHigh.unshift(vHigh);
if (aLow[LoLength-1] != null) aLow.pop();
aLow.unshift(vLow);
}
aHigh[0] = vHigh;
aLow[0] = vLow;
if (aHigh[HiLength-1] == null || aLow[LoLength-1] == null) return;
// get LSMA values and assign to arrays
var vHiLSMA = getLSMA(aHigh, HiLength);
var vLoLSMA = getLSMA(aLow, LoLength);
if (getBarState() == BARSTATE_NEWBAR) {
if (aHiLSMA[HiLength-1] != null) aHiLSMA.pop();
aHiLSMA.unshift(vHiLSMA);
if (aLoLSMA[LoLength-1] != null) aLoLSMA.pop();
aLoLSMA.unshift(vLoLSMA);
}
aHiLSMA[0] = vHiLSMA;
aLoLSMA[0] = vLoLSMA;
return new Array (aHiLSMA[HiOffset], aLoLSMA[LoOffset]);
}
// ------------------------------------
// Get a LSMA value
function getLSMA(aPrice, nLength) {
var Num1 = 0.0;
var Num2 = 0.0;
var SumBars = nLength * (nLength - 1) * 0.5;
var SumSqrBars = (nLength - 1) * nLength * (2 * nLength - 1) / 6;
var SumY = 0.0;
var Sum1 = 0.0;
var Sum2 = 0.0;
var Slope = 0.0;
var Intercept = 0.0;
for (i = 0; i < nLength; ++i) {
SumY += aPrice[i];
Sum1 += i * aPrice[i];
}
Sum2 = SumBars * SumY;
Num1 = nLength * Sum1 - Sum2;
Num2 = SumBars * SumBars - nLength * SumSqrBars;
if (Num2 != 0) Slope = Num1 / Num2;
Intercept = (SumY - Slope * SumBars) / nLength;
var LinearRegValue = Intercept + Slope * (nLength - 1);
return LinearRegValue;
}